Array#
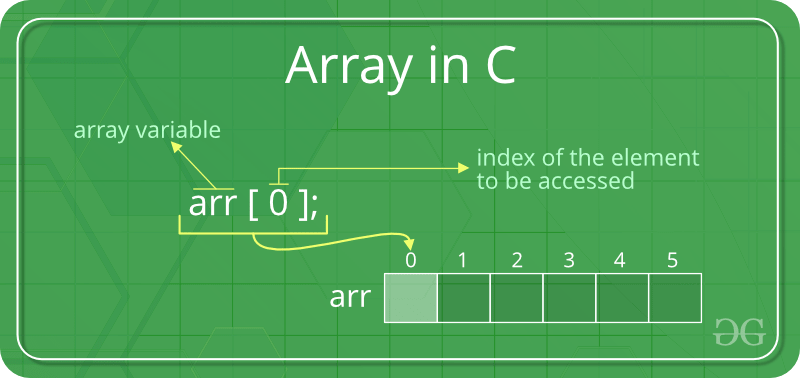
Definition
An array is a fundamental data structure that stores a collection of elements, such as numbers or strings, at contiguous memory locations. Each element in an array is identified by its index or position.
Use Cases
Storage & Access of Data
Implementing data structures
Facilitating efficient searching and sorting algorithms
Storing pixel values in image processing.
Managing large datasets in scientific computing.
Pros & Cons
Fast and constant-time
access to elements using their indices
Efficient memory allocation
as elements are stored sequentially.
Universal Support
Supported in most programming languages
Fixed size
in most languages (dynamic arrays can mitigate this).
Slow
Insertions and deletions can be slow as elements need to be shifted.
Memory Allocation
Wasting memory space if not fully utilized
Errors
Index out-of-bounds errors can occur if not handled properly
array
Syntax#
1. Initialize an array arr with a fixed size or dynamic sizing option.
2. Insert elements into arr.
3. Access and modify elements in arr.
4. Iterate through arr using loops.
5. Search for elements in arr.
6. Delete elements from arr.
7. Perform other operations as needed.
1#include <iostream>
2#include <array>
3
4int main() {
5 std::array<int, 3> arr; // Fixed-size array with size 3
6
7 // Insert elements
8 arr[0] = 10;
9 arr[1] = 20;
10 arr[2] = 30;
11
12 // Access and modify elements
13 arr[1] = 25;
14
15 // Iterate through arr
16 for (int i = 0; i < arr.size(); ++i) {
17 std::cout << arr[i] << " ";
18 }
19
20 return 0;
21}
1#include <iostream>
2#include <vector>
3
4int main() {
5 std::vector<int> arr; // Dynamic array
6
7 // Insert elements
8 arr.push_back(10);
9 arr.push_back(20);
10 arr.push_back(30);
11
12 // Access and modify elements
13 arr[1] = 25;
14
15 // Iterate through arr
16 for (int i = 0; i < arr.size(); ++i) {
17 std::cout << arr[i] << " ";
18 }
19
20 return 0;
21}
td::array is fixed-size and provides compile-time size checking.
C++ uses zero-based indexing.
C++ is known for its high performance, making it suitable for low-level and system programming.
std::array provides safety with fixed-size constraints.
Strong support for object-oriented programming (OOP) and generic programming.
Extensive standard library with various data structures and algorithms.
1arr = [] # Dynamic array
2
3# Insert elements
4arr.append(10)
5arr.append(20)
6arr.append(30)
7
8# Access and modify elements
9arr[1] = 25
10
11# Iterate through arr
12for element in arr:
13 print(element, end=' ')
Python uses dynamic arrays (lists) exclusively.
Lists can hold elements of different data types.
Python uses zero-based indexing.
Python is known for its simplicity and readability, making it a popular choice for beginners.
Lists are versatile and can easily hold different types of data.
Python offers a wide range of libraries for various tasks, such as data analysis and web development.
Dynamic typing allows for flexible and rapid development.
1public class ArrayExample {
2 public static void main(String[] args) {
3 int[] arr = new int[3]; // Fixed-size array
4
5 // Insert elements
6 arr[0] = 10;
7 arr[1] = 20;
8 arr[2] = 30;
9
10 // Access and modify elements
11 arr[1] = 25;
12
13 // Iterate through arr
14 for (int i = 0; i < arr.length; i++) {
15 System.out.print(arr[i] + " ");
16 }
17 }
18}
Java uses fixed-size arrays exclusively.
Arrays are declared with a specific size and cannot be resized.
Java uses zero-based indexing.
Java is platform-independent, thanks to the Java Virtual Machine (JVM), making it suitable for cross-platform development.
Strongly typed language with strict type checking, reducing runtime errors.
Robust and well-suited for large-scale applications and enterprise-level software.
Extensive standard library and a vast ecosystem of third-party libraries.
1fn main() {
2 let mut arr: Vec<i32> = Vec::new(); // Dynamic array
3
4 // Insert elements
5 arr.push(10);
6 arr.push(20);
7 arr.push(30);
8
9 // Access and modify elements
10 arr[1] = 25;
11
12 // Iterate through arr
13 for element in &arr {
14 print!("{} ", element);
15 }
16}
Rust uses dynamic arrays (Vec) exclusively.
Rust employs a strict ownership system and borrowing rules for memory safety.
Rust enforces zero-cost abstractions and guarantees memory safety without a garbage collector.
Rust excels in system programming, offering memory safety and low-level control.
The ownership system ensures thread safety and prevents common programming errors like null pointer dereferences.
Rust is suitable for building high-performance and reliable software, including operating systems and embedded systems.
It has a growing ecosystem of libraries and frameworks.
1package main
2
3import "fmt"
4
5func main() {
6 arr := []int{} // Dynamic array
7
8 // Insert elements
9 arr = append(arr, 10)
10 arr = append(arr, 20)
11 arr = append(arr, 30)
12
13 // Access and modify elements
14 arr[1] = 25
15
16 // Iterate through arr
17 for _, element := range arr {
18 fmt.Printf("%d ", element)
19 }
20}
Go uses dynamic arrays (slices) exclusively.
Slices are references to underlying arrays, making them efficient for passing subsets of data.
Go uses zero-based indexing.
Go is designed for simplicity, readability, and productivity, making it a popular choice for web and network programming.
Goroutines and channels enable concurrent programming with ease.
Garbage collection simplifies memory management for developers.
Go excels in building scalable and concurrent applications, such as web servers.
Output
An array of size three (3) is declared and instantiated. The data in element #1 is modified. A loop then prints each of the values from the array in order.
10 25 30