Data Types#
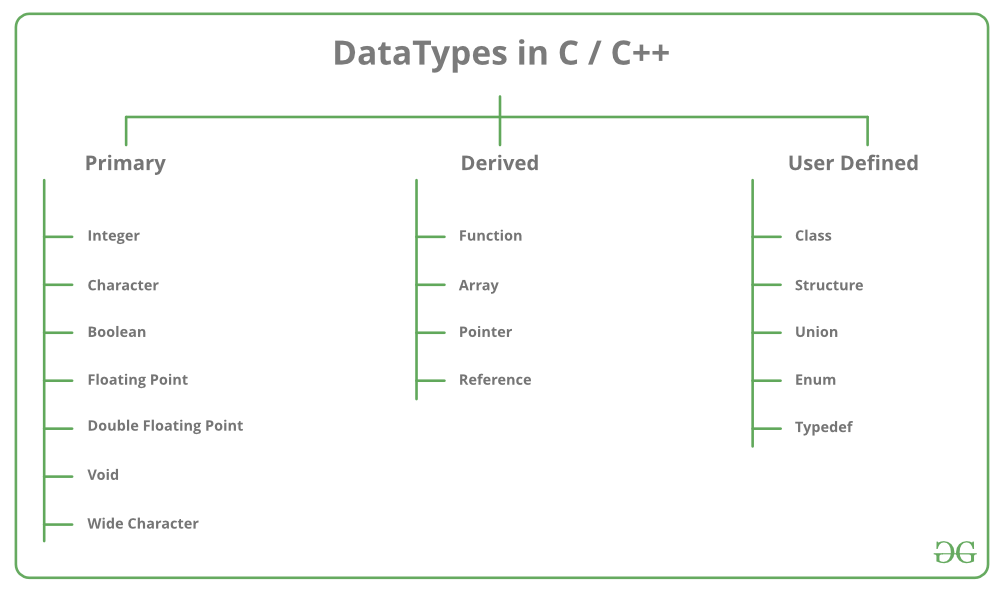
Overview#
Definition
Data types in programming languages represent the type of data that can be stored in variables. They define the size, memory allocation, and operations that can be performed on the data. Common data types include integers, floating-point numbers, characters, strings, booleans, and more complex types like arrays and structures.
Use Cases
Data types are essential for defining variables and data structures to hold different kinds of information in a program. They help enforce constraints on data and enable efficient memory management and manipulation.
Pros & Cons
Type Safety
Data types help catch type-related errors at compile-time, enhancing code reliability.
Memory Optimization
Choosing appropriate data types can optimize memory usage.
Performance
Properly selected data types improve the execution speed of programs.
Code Clarity
Explicit data types make code more readable and self-documenting.
Type Constraints
Incorrectly chosen data types can lead to unnecessary restrictions or potential errors.
Memory Overhead
In certain situations, using larger data types can lead to memory waste.
Conversion Overhead
Implicit type conversions can lead to performance overhead in some cases.
Syntax#
input length, width
area = length * width
print area
1#include <iostream>
2using namespace std;
3
4int main() {
5 double length, width;
6 cout << "Enter length: ";
7 cin >> length;
8 cout << "Enter width: ";
9 cin >> width;
10
11 double area = length * width;
12 cout << "Area of the rectangle: " << area << endl;
13
14 return 0;
15}
1length = float(input("Enter length: "))
2width = float(input("Enter width: "))
3
4area = length * width
5print("Area of the rectangle:", area)
1import java.util.Scanner;
2
3public class Main {
4 public static void main(String[] args) {
5 Scanner scanner = new Scanner(System.in);
6 double length, width;
7 System.out.print("Enter length: ");
8 length = scanner.nextDouble();
9 System.out.print("Enter width: ");
10 width = scanner.nextDouble();
11
12 double area = length * width;
13 System.out.println("Area of the rectangle: " + area);
14 }
15}
1use std::io;
2
3fn main() {
4 let mut input = String::new();
5 println!("Enter length: ");
6 io::stdin().read_line(&mut input).expect("Failed to read line");
7 let length: f64 = input.trim().parse().expect("Please enter a valid number");
8
9 input.clear();
10 println!("Enter width: ");
11 io::stdin().read_line(&mut input).expect("Failed to read line");
12 let width: f64 = input.trim().parse().expect("Please enter a valid number");
13
14 let area = length * width;
15 println!("Area of the rectangle: {}", area);
16}
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 var length, width float64
9
10 fmt.Print("Enter length: ")
11 fmt.Scan(&length)
12
13 fmt.Print("Enter width: ")
14 fmt.Scan(&width)
15
16 area := length * width
17 fmt.Println("Area of the rectangle:", area)
18}
Output
The code samples above calculate the
area
of a rectangle given its length and width. The user enters thelength
andwidth
, and the program performs the necessary calculations.Assume the user enters
length = 5
andwidth = 7
for all the examples.
C++ / Rust / Golang
Area of the rectangle: 35
Python / Java
Area of the rectangle: 35.0
Note: the difference in each output is due directly to the nature of integer division within each given language.
Data Types of Various Languages#
Many of the following overlap between languages while others, are unique to a given language. Implementations are in C++.
Integer Types
int
- Used to represent integers.
int age = 25;
short
- Used to represent short integers.
short quantity = 10;
long
- Used to represent long integers.
long population = 1000000;
long long
- Used to represent very long integers.
long long distance = 10000000000LL;
unsigned int
- Used to represent positive integers.
unsigned int count = 100;
Floating-Point Types
float
- Used to represent floating-point numbers with single precision.
float weight = 65.5f;
double
- Used to represent floating-point numbers with double precision.
double pi = 3.14159;
long double
- Used to represent floating-point numbers with extended precision.
long double value = 1234.56789L;
Character Types
char
- Used to represent single characters.
char grade = 'A';
wchar_t
- Used to represent wide characters.
wchar_t symbol = L'€';
Boolean Type
bool
- Used to represent boolean values (true or false).
bool isValid = true;