Functions#
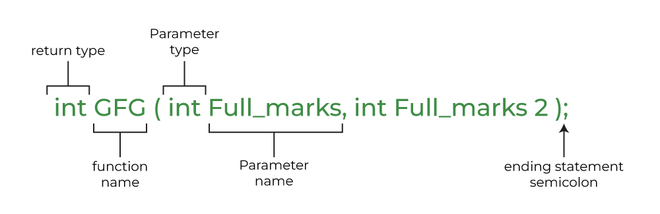
Definition#
Definition
A function is a self-contained block of code that performs a specific task and can be called or invoked from other parts of a program. Functions are essential for code modularity and reusability.
Use Cases
Encapsulate a task or logic
Promote code reuse
Improve code readability and maintainability
Pros & Cons
Modularity
Functions break down complex tasks into smaller, manageable pieces.
Reusability
Functions can be used in different parts of a program.
Readability
Well-named functions make code easier to understand.
Debugging
Isolating functions helps in pinpointing and fixing issues.
Scoping
Functions define variable scopes, reducing naming conflicts.
Overhead
Functions may introduce a slight overhead in terms of memory and performance.
Complexity
Overuse of functions can lead to code that’s hard to follow.
Maintainability
Poorly designed functions can hinder rather than help.
Function Syntax#
Function square(number)
Result = number * number
Return Result
End Function
1#include <iostream>
2
3int square(int number) {
4 int result = number * number;
5 return result;
6}
7
8int main() {
9 int x = 5;
10 int squared = square(x);
11 std::cout << "Square of " << x << " is " << squared << std::endl;
12 return 0;
13}
C++ provides low-level memory control, allowing you to manage memory directly.
Function overloading: You can define multiple functions with the same name but different parameter lists.
Strong type system with a high degree of control over data types.
Supports both pass-by-value and pass-by-reference parameter passing.
High performance: C++ is known for its efficiency and is often used in systems programming and game development.
Extensive standard library (STL) for various data structures and algorithms.
Compatibility with C: You can use C libraries and code seamlessly in C++.
1def square(number):
2 result = number * number
3 return result
4
5x = 5
6squared = square(x)
7print(f"Square of {x} is {squared}")
Dynamic typing: Variable types are determined at runtime, making code more flexible but potentially error-prone.
Indentation-based block structure: Python uses indentation for code blocks, enhancing readability but requiring strict formatting.
Interpreted language: Python code is executed line by line, which may lead to slower performance compared to compiled languages.
Simplicity and readability: Python’s clean syntax makes it easy to learn and read.
Rich standard library: Python offers extensive libraries for various tasks, including web development, data analysis, and machine learning.
Strong community and ecosystem support.
1public class Main {
2 public static int square(int number) {
3 int result = number * number;
4 return result;
5 }
6
7 public static void main(String[] args) {
8 int x = 5;
9 int squared = square(x);
10 System.out.println("Square of " + x + " is " + squared);
11 }
12}
Strongly typed: Java enforces strict type checking, reducing type-related errors.
Object-oriented: Java is known for its object-oriented programming (OOP) paradigm.
Platform independence: Java code runs on the Java Virtual Machine (JVM), making it highly portable.
Write Once, Run Anywhere (WORA): Java’s platform independence allows you to run code on multiple platforms without modification.
Robust and secure: Java emphasizes error handling and security features.
Extensive libraries and frameworks: Java has a vast ecosystem of libraries and frameworks for various applications.
1fn square(number: i32) -> i32 {
2 let result = number * number;
3 result
4}
5
6fn main() {
7 let x = 5;
8 let squared = square(x);
9 println!("Square of {} is {}", x, squared);
10}
Ownership system: Rust introduces concepts like ownership, borrowing, and lifetimes to ensure memory safety without a garbage collector.
Pattern matching: Rust provides powerful pattern matching capabilities.
Concurrency without data races: Rust’s ownership system prevents data races in concurrent code.
Memory safety: Rust is designed to eliminate common memory-related bugs like null pointer dereferences and buffer overflows.
Performance: Rust offers performance comparable to C and C++ while ensuring safety.
System-level programming: Rust is well-suited for systems programming, embedded systems, and writing safe low-level code.
1package main
2
3import "fmt"
4
5func square(number int) int {
6 result := number * number
7 return result
8}
9
10func main() {
11 x := 5
12 squared := square(x)
13 fmt.Printf("Square of %d is %d\n", x, squared)
14}
Simplicity and minimalism: Go emphasizes simplicity and readability with a minimalistic syntax.
Goroutines: Go uses lightweight threads called goroutines for concurrency.
No classes or inheritance: Go uses a different approach to achieve abstraction and code organization.
Concurrency: Goroutines and channels make concurrent programming more accessible and safe.
Compilation speed: Go compiles quickly, aiding fast development cycles.
Web development: Go is popular for building web servers and microservices due to its efficiency and simplicity.
Output
Calculate the square of a number
Congratulations! You passed the exam.
Compare & Contrast#
Aspect |
C++ |
Python |
Java |
Rust |
Golang |
---|---|---|---|---|---|
Syntax for Functions |
Traditional |
Def/Return |
Declaration |
fn/-> |
func/return |
Strong Typing |
Yes |
Dynamic |
Strong |
Strong |
Static |
Memory Management |
Manual |
Automatic |
Automatic |
Automatic |
Automatic |
Concurrency Support |
Yes |
Yes |
Yes |
Yes |
Yes |
Safety (No Null/Segfaults) |
Limited |
Yes |
Yes |
Yes |
Yes |