Iteration Statements#
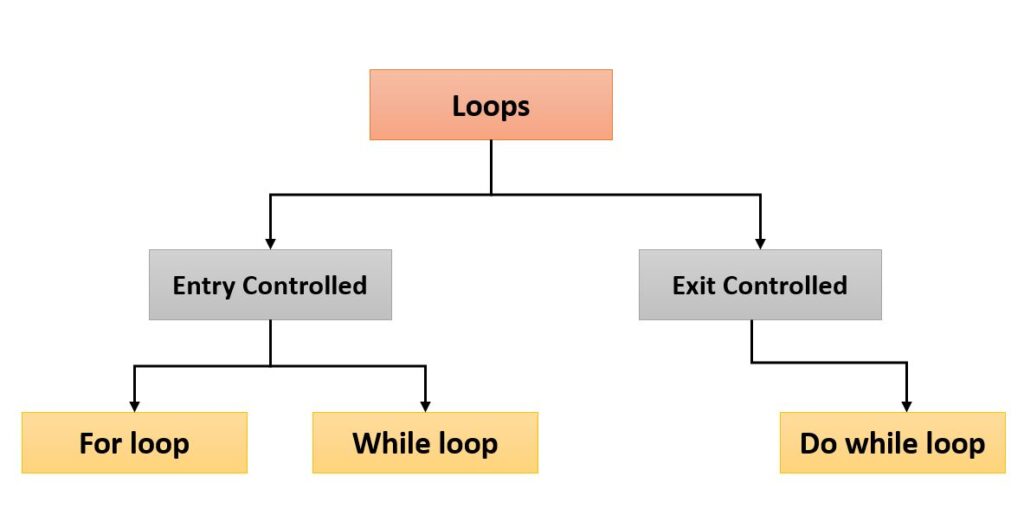
Loops#
Entry Controlled#
for
loop#
The for loop is used when the number of iterations is known in advance. It consists of three parts: initialization, condition, and iteration.
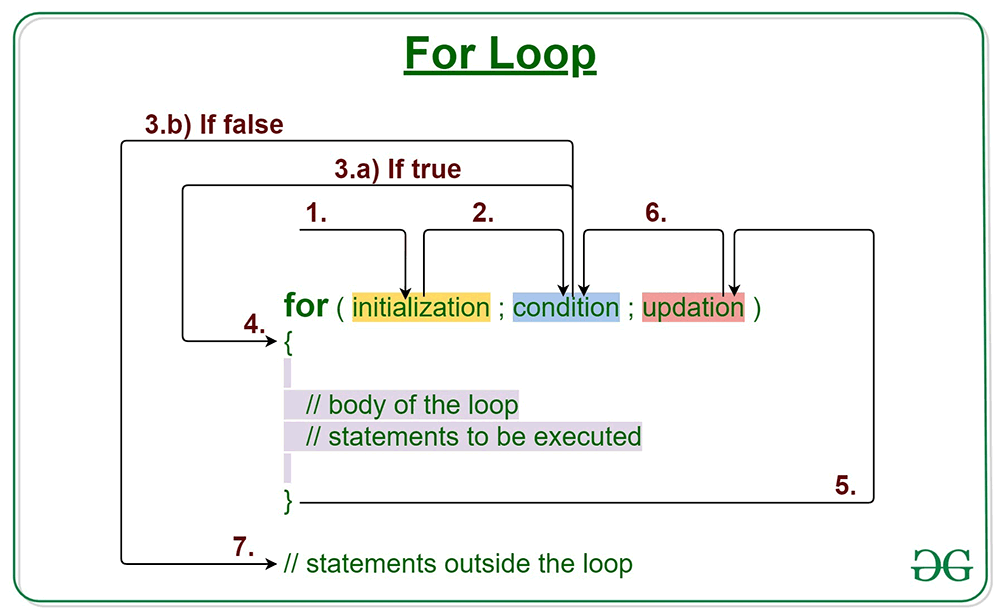
Fig. 1 for
loop#
// Syntax
for (initialization; condition; iteration) {
// Code block to be executed repeatedly
}
// Example
// C++ program to illustrate If statement
#include <iostream>
using namespace std;
int main()
{
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
}
// Output
0
1
2
3
4
The loop initializes the variable i
to 0
, checks the condition i < 5
, executes the code block, increments i
by 1
, and repeats the process until the condition is no longer true
.
while
loop#
The while loop is used when the number of iterations is not known in advance. It repeats a block of code as long as a specified condition is true.
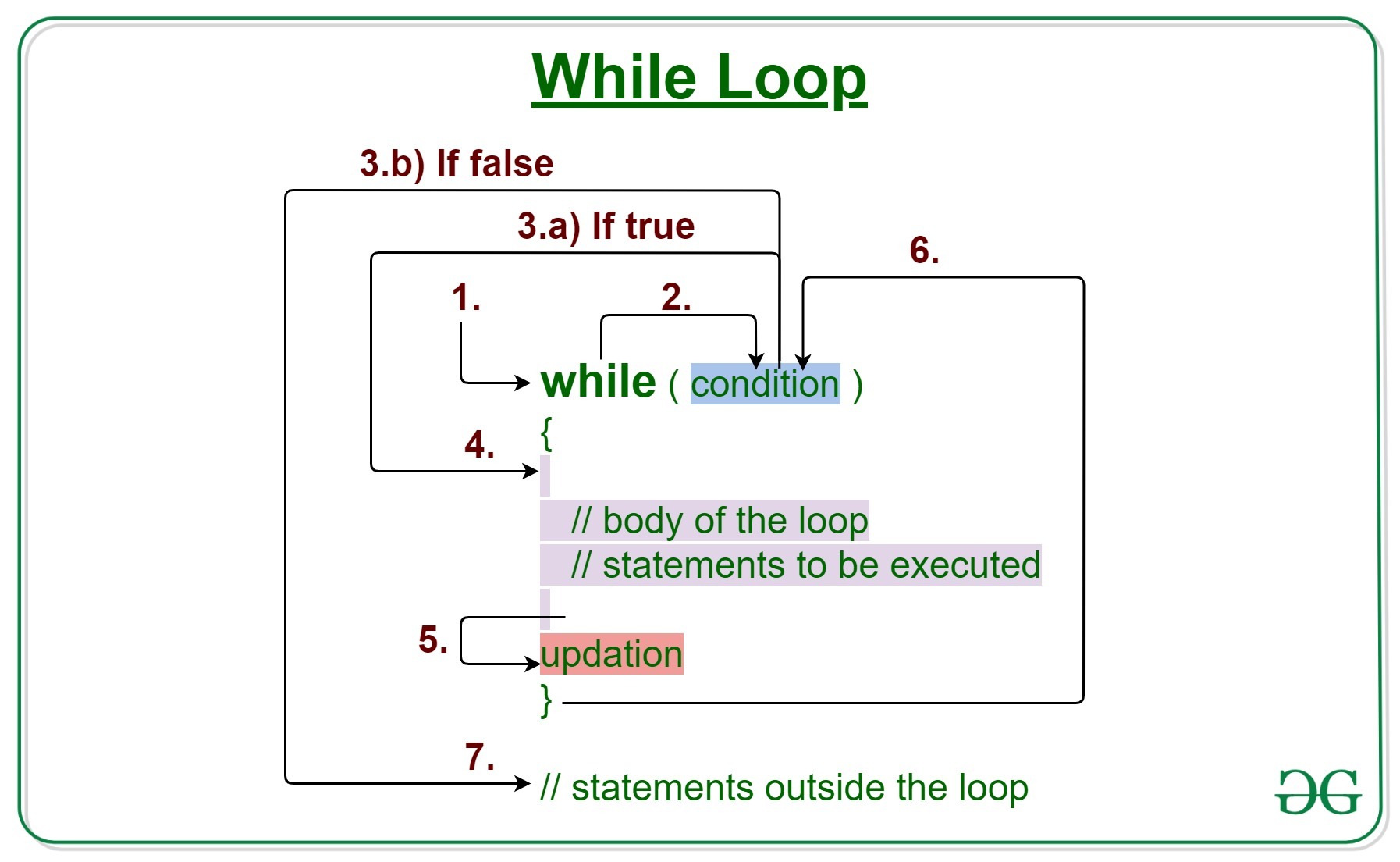
Fig. 2 while
loop#
// Syntax
while (condition) {
// Code block to be executed repeatedly
// Condition should eventually become false to exit the loop
}
// Example
// C++ program to illustrate If statement
#include <iostream>
using namespace std;
int main()
{
int i = 0;
while (i < 5) {
cout << i << endl;
i++;
}
}
// Output
0
1
2
3
4
The loop checks the condition i < 5 before each iteration. If the condition is true, it executes the code block, increments i by 1, and repeats the process until the condition becomes false.
Exit Controlled#
do while
#
The do-while loop is similar to the while loop, but it guarantees that the code block is executed at least once before checking the condition.
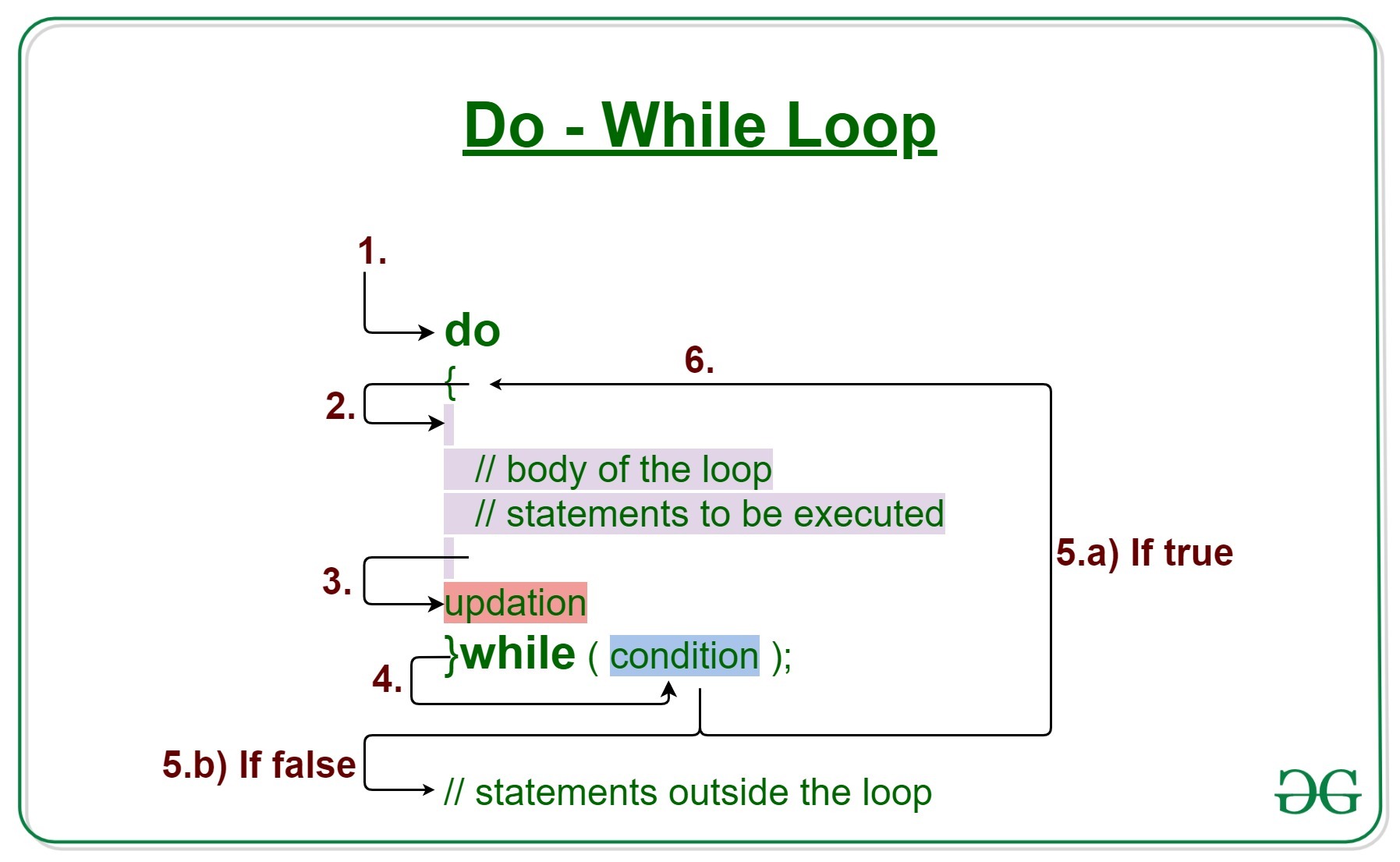
Fig. 3 do-while
loop#
// Syntax
do {
// Code block to be executed repeatedly
// Condition should eventually become false to exit the loop
} while (condition);
// Example
// C++ program to illustrate If statement
#include <iostream>
using namespace std;
int main()
{
int i = 0;
do {
cout << i << endl;
i++;
} while (i < 5);
}
// Output
0
1
2
3
4
The loop executes the code block at least once because the condition i < 5 is checked after the first iteration. If the condition is true, it repeats the process until the condition becomes false.